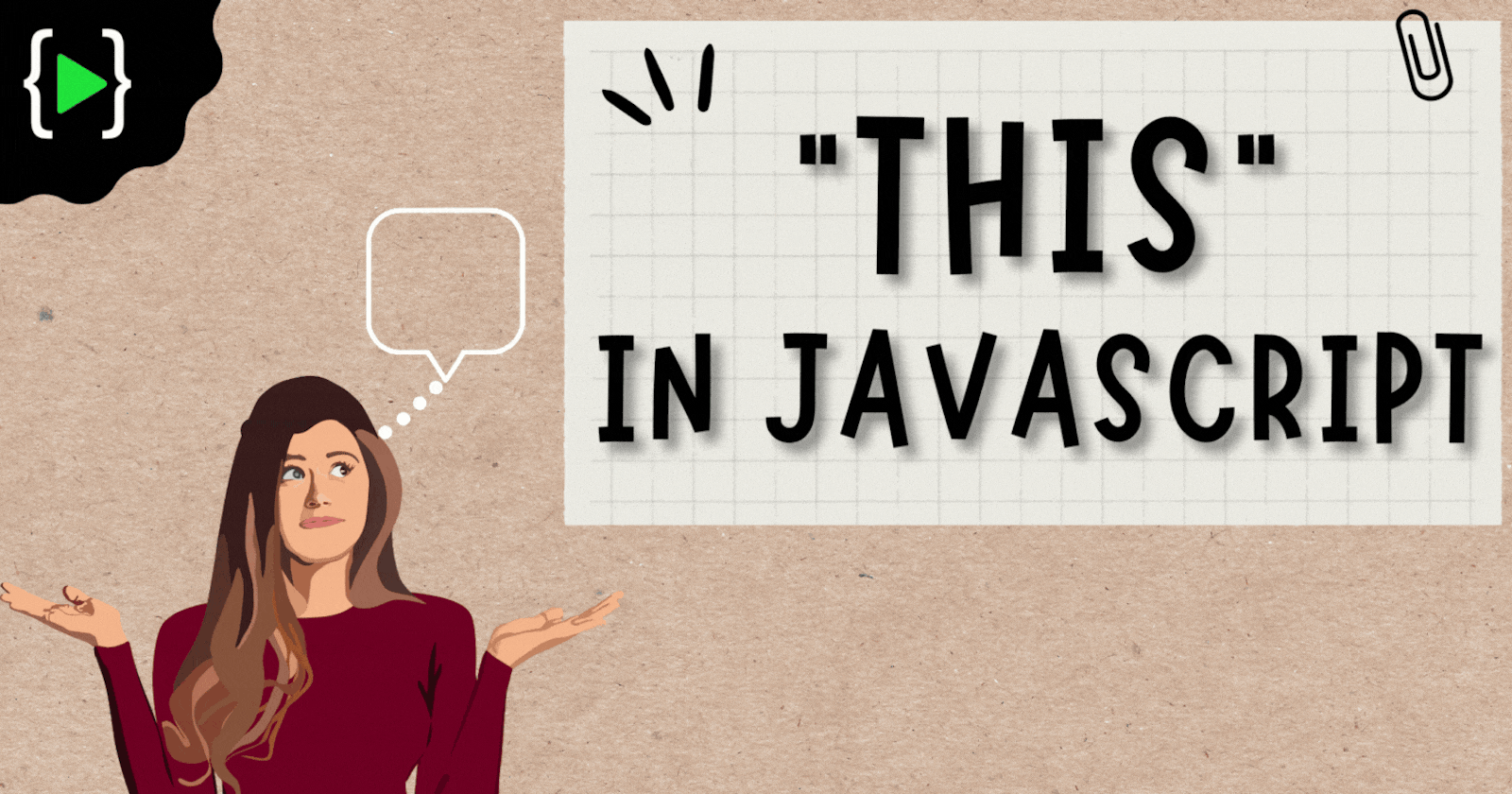
JavaScript "this" Keyword
How the ‘this’ keyword changes when used in different contexts
Hello everyone👋🏻,
In this article, you will learn about JavaScript's this
keyword with the help of examples.this
keyword is one of the most widely used and yet confusing keyword in JavaScript. 😇
What is "this" in JavaScript?🤔
In JavaScript, the
this
keyword refers to the object where it is called.
this
points to a particular object. Now, which is that object depends on how a function that includes the 'this' keyword is being called.
The “this” keyword in JavaScript(JS) is a reserved keyword meant to help you target certain objects in JavaScript.
To be more specific, it’s meant to help you target the current object.
"this" Inside Global Context🌍
What happens if you reference this in the global context (i.e., not inside any function)?
console.log(this);
As that code demonstrates, this in the global context refers to the window object.
Look at the following code and the subsequent outputs:
this.greet = "Hello World!";
window.greet = "Hello World!";
console.log(this.greet );
console.log(window.greet );
console.log(greet );
So what’s going on here? 🤔 The first two lines are doing the exact same thing — creating a global variable called
“greet”
and setting its value to “Hello World!”
This fact is demonstrated by the three console messages that follow, all showing the same result
.
"this" Inside Function Context ⚡
If a function that includes 'this'
keyword, is called from the global scope then this will point to the window object. Learn about global and local scope here.
var myNum = 100;
function WhatIsThis() {
var myNum = 200;
console.log("myNum = " + myNum); // 200
console.log("this.myNum= " + this.myNum); // 100
}
WhatIsThis(); // inferred as window.WhatIsThis()
In the above example,
- the function
WhatIsThis()
is called from the global scope. - The global scope means in the context of the
window object
. - We can optionally call it like a
window.WhatIsThis()
. - So in the above example,
this
keyword in theWhatIsThis()
function will refer to thewindow object
. - So,
this.myNum
will return100
. However, if you accessmyNum
without this then it will refer to the localmyNum
variable defined inWhatIsThis()
function.
The following figure illustrates the above example.
As you can see, nothing’s changed from the global context (i.e. this still references the window object).
"this" Inside Object Method💡
When this
is used inside an object's method
, this refers to the object it lies within.
For example,
const student = {
name : 'Chhakuli',
age: 21,
// this inside method
// this refers to the object itself
greet() {
console.log(this);
console.log(this.name);
}
}
student.greet();
Output:
In the above example,
this
refers to thestudent
object.
"this" Inside Inner Function 💠
When you access this
inside an inner function (inside a method)
, this refers to the global object
.
For example,
const student = {
name : 'Chhakuli',
age: 21,
// this inside method
// this refers to the object itself
greet() {
console.log(this); // {name: "Chhakuli", age ...}
console.log(this.age); // 21
// inner function
function innerFunc() {
// this refers to the global object
console.log(this); // Window { ... }
console.log(this.age); // undefined
}
innerFunc();
}
}
student.greet();
Here, this inside
innerFunc()
refers to the global object becauseinnerFunc()
is inside a method.However,
this.age
outside innerFunc() refers to the student object.
this Inside Function with Strict Modet😬
When this
is used in a function with strict mode
, this is undefined.
For example,
'use strict';
this.name = 'Chhakuli';
function greet() {
// this refers to undefined
console.log(this);
}
greet(); // undefined
Note: When using this inside a function with strict mode, you can use JavaScript Function call().
For example,
'use strict';
this.name = 'Chhakuli';
function greet() {
console.log(this.name);
}
greet.call(this); //Chhakuli
When you pass
this
with thecall()
function,greet()
is treated as the method ofthis
object (global object in this case).
Conclusion🙇♂️
If nothing else, this post should demonstrate that using this can be a useful shortcut. But all the while, keep in mind how the context can change the value of this
as well as how it behaves in strict mode
.
I’ve only scratched the surface of this topic, so here are some further post discussing the this
keyword: 😄